- Published on
Swift Ranges
- Authors
- Name
- Wayne Dahlberg
- @waynedahlberg
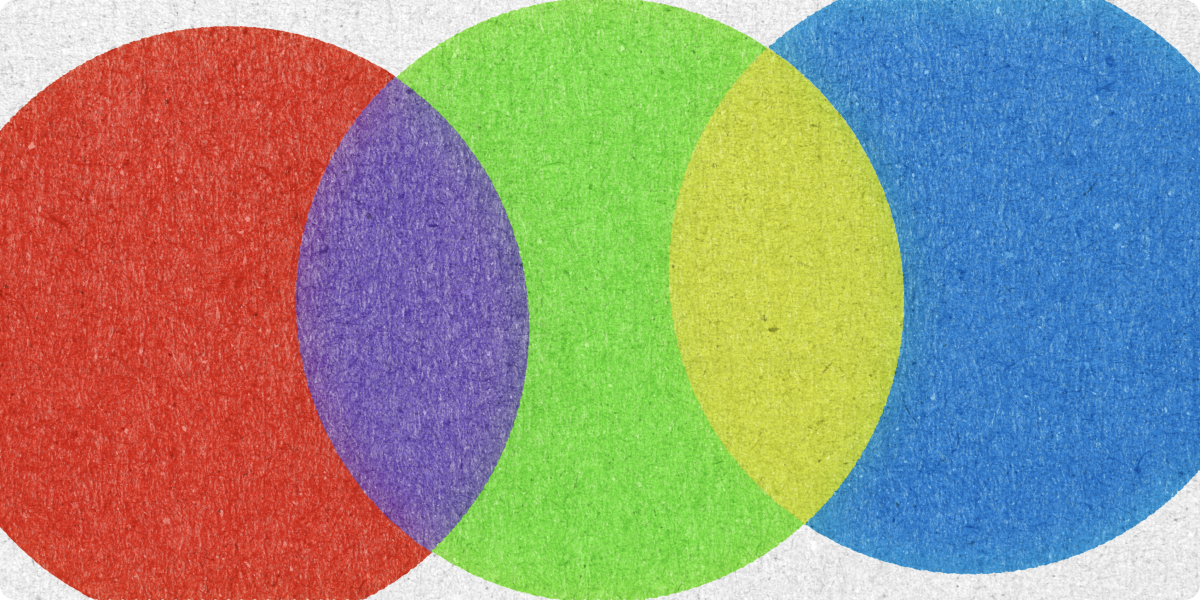
Ranges are generic Swift Structures for selecting a range, or a limited subset, of an existing type. Ranges are used commonly for identifying elements between the lowest and highest values in a set.
This is a another post in a series intended as a personal growth exercise. As I learn and digest new things, I want to write about them to solidify my understanding.
Kinds of Ranges
Swift gives us two ways of creating ranges. Half-Open range, and Closed Range.
Half-Open Range
The Half-Open range operator is declared with ..<
between a numeric pair.
Using a Range
instance, you can quickly check to see if a value is contained in a particular range of values. This creates a range up to but not including the upper bound value.
let underFive = 0.0..<5.0
underFive.contains(3.14) // true
underFive.contains(6.28) // false
underFive.contains(5.0) // false (upper bound value not included)
Range instances can represent an empty interval, unlike Closed Range(see below.)
let empty = 0.0..<0.0
empty.contains(0.0) // false
empty.isEmpty // true
Range can also be used to specify a collection of consecutive, strideable values, usually Integers. It can also be used in for-in
loops or with any sequence or collection method. The results of the range include all values up to, but not including the upperBound.
for n in 3..<5 {
print(n)
}
// prints "3"
// prints "4"
// does NOT print "5"
Ranges help in Switch statements, using them for your cases. Consider the following:
let score = 92
switch score {
case 0..<50:
print("Fail")
case 50..<90:
print("Pass")
default:
print("Excellent")
} // prints "Excellent"
Closed Range
A Closed range operator is declared with ...
between a pair of numeric values. The left value being the lowerBound
and the right value being upperBound
. The Closed Range includes the upperBound value.
let range : ClosedRange = 0...5
print(range) // 0...5
print(range.first) // Optional(0)
print(range.last) // Optional(10)
for index in range {
print(index) // prints consecutivly, values from 0 to 5
}
Inspecting a Range
Here are just a few of the operations you can call on a range instance in Swift. There are many more in Apple's Documentation for Range.
var isEmpty: Bool
Returns a Boolean value indicating whether the range contains no elements.
var count: Int
Returns the number of elements in the collection of values.
let lowerBound: Bound
Returns the range's lower bound
let upperBound: Bound
Returns the range's upper bound
That's it for this quick post. See you in the next one.